https://www.acmicpc.net/problem/2606
2606번: 바이러스
첫째 줄에는 컴퓨터의 수가 주어진다. 컴퓨터의 수는 100 이하이고 각 컴퓨터에는 1번 부터 차례대로 번호가 매겨진다. 둘째 줄에는 네트워크 상에서 직접 연결되어 있는 컴퓨터 쌍의 수가 주어
www.acmicpc.net
문제
신종 바이러스인 웜 바이러스는 네트워크를 통해 전파된다. 한 컴퓨터가 웜 바이러스에 걸리면 그 컴퓨터와 네트워크 상에서 연결되어 있는 모든 컴퓨터는 웜 바이러스에 걸리게 된다.
예를 들어 7대의 컴퓨터가 <그림 1>과 같이 네트워크 상에서 연결되어 있다고 하자. 1번 컴퓨터가 웜 바이러스에 걸리면 웜 바이러스는 2번과 5번 컴퓨터를 거쳐 3번과 6번 컴퓨터까지 전파되어 2, 3, 5, 6 네 대의 컴퓨터는 웜 바이러스에 걸리게 된다. 하지만 4번과 7번 컴퓨터는 1번 컴퓨터와 네트워크상에서 연결되어 있지 않기 때문에 영향을 받지 않는다.
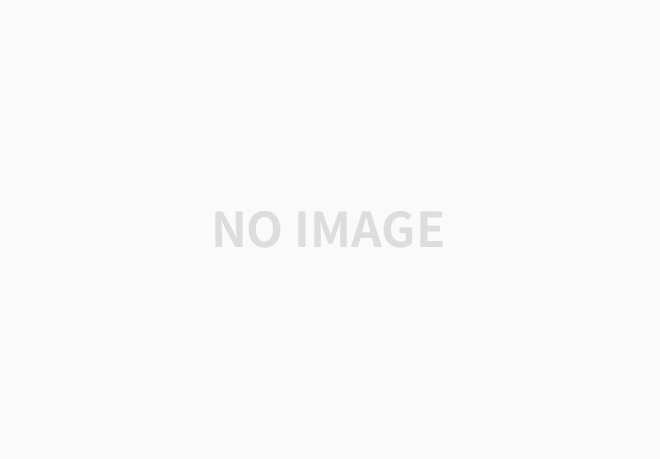
어느 날 1번 컴퓨터가 웜 바이러스에 걸렸다. 컴퓨터의 수와 네트워크 상에서 서로 연결되어 있는 정보가 주어질 때, 1번 컴퓨터를 통해 웜 바이러스에 걸리게 되는 컴퓨터의 수를 출력하는 프로그램을 작성하시오.
입력
첫째 줄에는 컴퓨터의 수가 주어진다. 컴퓨터의 수는 100 이하이고 각 컴퓨터에는 1번부터 차례대로 번호가 매겨진다. 둘째 줄에는 네트워크 상에서 직접 연결되어 있는 컴퓨터 쌍의 수가 주어진다. 이어서 그 수만큼 한 줄에 한 쌍씩 네트워크 상에서 직접 연결되어 있는 컴퓨터의 번호 쌍이 주어진다.
출력
1번 컴퓨터가 웜 바이러스에 걸렸을 때, 1번 컴퓨터를 통해 웜 바이러스에 걸리게 되는 컴퓨터의 수를 첫째 줄에 출력한다.
예제 입력 1 복사
7
6
1 2
2 3
1 5
5 2
5 6
4 7
예제 출력 1 복사
4
문제 풀이
1. 입력을 받는다. 무방향 그래프를 ArrayList를 이용하여 구현하였다.
2. BFS로 접근했다. map[]을 만들어 방문을 확인했다.
3. 방문을 안했다면 방문 처리를 해주고 Queue에 값을 추가하고 cnt++(연결되어 있는 컴퓨터 수)를 늘려줬다.
4. 방문을 했다면 continue를 사용하여 다음 값을 확인했다.
BFS 사용
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
|
import java.io.*;
import java.util.*;
public class Main {
static ArrayList<Integer> arr[];
static int map[];
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
int N = Integer.parseInt(st.nextToken());
st = new StringTokenizer(br.readLine());
int T = Integer.parseInt(st.nextToken());
map = new int[N+1];
arr = new ArrayList[N+1];
for(int i = 1 ; i < N+1 ; i++) { // 열결 상태 저장
arr[i] = new ArrayList<>();
}
for(int i = 0 ; i < T ; i++) { // 값 저장
st = new StringTokenizer(br.readLine());
int from = Integer.parseInt(st.nextToken());
int to = Integer.parseInt(st.nextToken());
arr[from].add(to);
arr[to].add(from);
}
Queue<Integer> q = new LinkedList<>();
q.add(1); // Queue에 1을 추가
map[1] = 1; // 1은 방문 처리를 한다.
int cnt =0 ;
while(!q.isEmpty()) { // queue가 비어있지 않다면
int now = q.poll(); // queue의 첫 번째 값을 가져오고 queue 안에서는 없앤다
for(int i = 0; i < arr[now].size() ; i++) {
int next = arr[now].get(i); // arr index안의 값을 i의 값 순서대로 가져온다
if(map[next] != 1) { // 방문하지 않았다면
map[next] = 1; // 방문 처리를 하고
q.add(next); // queue에 값을 추가한다
cnt++; // 1과 연결된 컴퓨터를 찾았으므로 1을 더해준다
}
}
}
System.out.println(cnt);
}
}
|
cs |
DFS 사용
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
import java.util.*;
import java.io.*;
public class Main {
static int computerN;
static int connectedN;
static int count = 0;
static boolean[] isVisit;
static ArrayList<Integer> arr[];
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
computerN = Integer.parseInt(st.nextToken());
st = new StringTokenizer(br.readLine());
connectedN = Integer.parseInt(st.nextToken());
arr = new ArrayList[computerN + 1];
isVisit = new boolean[computerN + 1];
for (int i = 0; i < arr.length; i++) {
arr[i] = new ArrayList<>();
}
for (int i = 0; i < connectedN; i++) {
st = new StringTokenizer(br.readLine());
int a = Integer.parseInt(st.nextToken());
int b = Integer.parseInt(st.nextToken());
arr[a].add(b);
arr[b].add(a);
}
for (int i = 0; i < arr.length; i++) {
Collections.sort(arr[i]);
}
dfs(1);
System.out.println(count);
}
public static void dfs(int index) {
isVisit[index] = true;
for (int i: arr[index]) {
if(!isVisit[i]) {
dfs(i);
count++;
}
}
}
}
|
cs |
'코딩 문제 > 백준 [ Java ]' 카테고리의 다른 글
[ 백준 15654 / Java ] N과 M (5) (0) | 2022.01.29 |
---|---|
[ 백준 10026 / Java ] 적록색약 (0) | 2022.01.28 |
[ 백준 15652 / Java ] N과 M (4) (0) | 2022.01.27 |
[ 백준 15651 / Java ]N과 M (3) (0) | 2022.01.27 |
[ 백준 2108 / Java ] 통계학 (0) | 2022.01.26 |
댓글